학생 성적을 입력받아 출력하는 것입니다.
연결리스트를 사용합니다.
- 요구
- 음수 값이 들어오면 입력을 종료 - 모든 학생의 점수를 출력한다. |
- 소스코드
#define _CRT_SECURE_NO_WARNINGS #include<stdio.h> #include<string.h> #include<stdlib.h> struct linked_list { int score; struct linked_list* next; }; typedef struct linked_list NODE; typedef NODE* LINK; LINK createNode(int score); LINK append(LINK head, LINK newNode); int printList(LINK head); //연결 리스트의 모든 노드 출력 함수 int main(void) { int score; LINK head = NULL; //연결 리스트의 헤드로 사용 LINK newNode; //현재 새로이 생성된 노드를 가리키는 포인터 printf("점수를 입력하고 Enter를 누르세요.\n"); printf("(입력을 종료는 음수입니다)\n"); printf(">> "); scanf("%d", &score); while (score >= 0) { newNode = createNode(score); //노드 동적 할당 if (newNode == NULL) { printf("동적메모리 할당에 문제가 있습니다.\n"); exit(1); } head = append(head, newNode); scanf("%d", &score); } printList(head); //연결 리스트 모두 출력 return 0; } LINK createNode(int score) { //노드를 생성하는 함수 LINK newNode; //새로 생성되는 노드의 주소를 저장할 변수 newNode를 선언 newNode = (LINK)malloc(sizeof(NODE)); if (newNode == NULL) { printf("노드 생성을 위한 메모리 할당에 문제가 있습니다.\n"); return NULL; } newNode->score = score; newNode->next = NULL; return newNode; } LINK append(LINK head, LINK newNode) { //newNode 노드를 연결 리스트에 추가하는 함수 LINK cur = NULL; LINK nextNode = head; if (head == NULL) { //연결리스트의 노드가 하나도 없는 경우 head = newNode; //추가하려는 노드가 head가 됨 return head; } while (newNode->score > nextNode->score) { //삽입 위치까지 이동 if (nextNode->next != NULL) { // 다음 멤버가 있을때 cur = nextNode; nextNode = nextNode->next; } else { // 맨 끝에 추가 nextNode->next = newNode; return head; } } newNode->next = nextNode; // 삽입 위치 찾음 if (cur != NULL) cur->next = newNode; //중간에 추가 else head = newNode; //맨앞에 추가할때 return head; } int printList(LINK head) { //연결 리스트 모두 출력하는 함수 int cnt = 0; //방문한 노드의 수를 저장 LINK nextNode = head; while (nextNode != NULL) { printf("%3d번째 학생의 성적: %d\n", ++cnt, nextNode->score); nextNode = nextNode->next; } return cnt; } |
- 결과화면
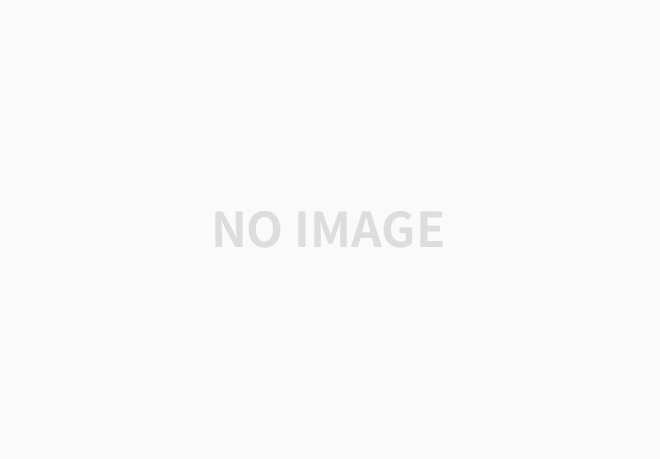
도움이 되었기를 바랍니다.
감사합니다.